Meshes
This tutorial walks through the basics of MCUT, showing how to define a mesh and iterate through its vertices and faces.
A mesh represents a two-manifold surface, where each edge is incident to one or two faces.
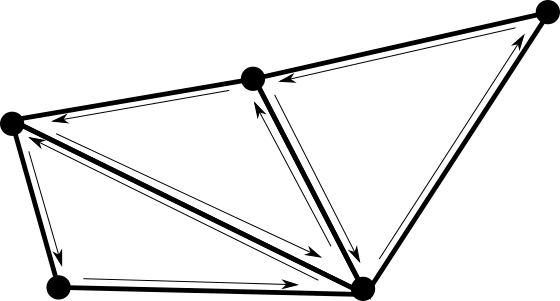
An example of a 2-manifold mesh.
Meshes in MCUT are represented as simple arrays of builtin C/C++ data types. In particular, users are permitted to use three C/C++ types when specifying vertex coordinates. These are float
and double
.
32-bit float
vertex arrays¶
The following is an example of how to define a mesh using the float
data type to represent vertex positions:
McFloat pMeshVertices[] = { // xyz|xyz|x...
-10.f, -5.f, 0.f, // vertex 0
-10.f, 5.f, 0.f, // vertex 1
10.f, 5.f, 0.f,
10.f, -5.f, 0.f,
5.f, 0.f, 0.f,
0.f, -5.f, 0.f,
-5.f, 0.f, 0.f,
-10.f, -10.f, 0.f,
10.f, -10.f, 0.f,
15.f, 5.f, 0.f,
15.f, -10.f, 0.f
};
McUint32 pMeshFaceIndices[] = {
0, 6, 5, 4, 3, 2, 1, // face 0
0, 7, 8, 3, 4, 5, 6, // face 1
8, 10, 9, 2, 3 // face 2
};
McFaceSize pMeshFaceSizes[] = {7, 7, 5};
McUint32 numMeshVertices = 11;
McUint32 numMeshFaces = 3;
64-bit double
vertex arrays¶
Using the same mesh example, the following except shows how to specify vertices as an array of double
:
McDouble pMeshVertices[] = {
-10.0, -5.0, 0.0,
-10.0, 5.0, 0.0,
10.0, 5.0, 0.0,
10.0, -5.0, 0.0,
5.0, 0.0, 0.0,
0.0, -5.0, 0.0,
-5.0, 0.0, 0.0,
-10.0, -10.0, 0.0,
10.0, -10.0, 0.0,
15.0, 5.0, 0.0,
15.0, -10.0, 0.0
};
Accessing mesh elements¶
As a simple demonstration of the mesh data structure defined above, let us iterate through the vertices and faces of the mesh.
// vertices
for (McUint32 i = 0; i < numMeshVertices; ++i) {
// assuming float
McFloat x = pMeshVertices[i*3 +0];
McFloat y = pMeshVertices[i*3 +1];
McFloat z = pMeshVertices[i*3 +2];
printf("vertex: %f %f %f\n", x, y, z);
}
// faces
McUint32 base = 0;
for(McInt32 i = 0; i < numMeshFaces; ++i){
McUint32 numFaceVertices = pMeshFaceSizes[i];
printf("face %d (%hu vertices):", i, numFaceVertices);
for(McUint32 j = 0; j < numFaceVertices; ++j) {
printf(" %u", pMeshFaceIndices[base+j]);
}
printf("\n");
base += numFaceVertices;
}